When I started learning programming, it was with the headaches that come with C. Back then, even storing a string in a struct made me think about things like, "How much memory do I need to allocate?" After that came Java, where everything changed. Object-Oriented Programming presented itself as a much easier alternative to understand and manage, and Java with its garbage collector greatly accelerated the development process, preventing me from algorithmically thinking about how to allocate and free memory dynamically as software requirements became more complex.
Feeling more comfortable with Vanilla Java (or as we say in Chile, "Java pela’o"), I started learning Java Spring Framework 5 (with Spring Boot), finally being able to develop more robust web applications. However, it always seemed to me that a lot of boilerplate code was necessary for an application to be functional.
When I started learning Ruby on Rails (RoR), for me, there was a before and after in web application development.

RoR is an open-source web development framework, written in Ruby, that follows the MVC (Model-View-Controller) architecture. It is incredibly fast in terms of getting something functional up and running. You can generate an application with a single command (rails new application_name), and you can also generate a CRUD with another command. For example, if I wanted to create a CRUD for blog articles, I would use one of Rails' generators called scaffold (meaning scaffolding in English, implying that it helps you build the framework your application will need in the future):
rails generate scaffold article title:string body:text
This command, "in human terms," would be something like, "Rails, I want you to generate the necessary code for a CRUD of the class Article, with the attributes 'title' of type varchar in the database, and 'body' of type text in the database." Specifically, this command generates a series of files within the file structure that Rails uses: Model, View, Controller, and Migration (a migration is a file that makes changes to the database programmatically, meaning that using Ruby you can add database statements without knowing its query language, such as SQL). A CRUD created with Rails' scaffold looks like this:
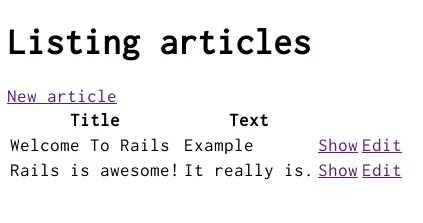
While it is very simple, it has all the necessary functionality to start with an MVP. And now, with this in mind, knowing that it's possible to literally generate a basic web page with a single command, can you imagine why Rails is considered a startup technology?
There are quite a few articles that discuss this topic. Some recommended ones are: "Why Ruby on Rails is Still a Strong Choice for Startups in 2020" — Hatchpad, "8 Startups That Owe Their Success to Ruby on Rails," and "Which startups are using Ruby on Rails now?". Although the last one is from 2018, it shows graphs (and links to graphs updated to 2020) about the trend in Rails usage, and the famous or high-traffic sites that use Rails (including Slideshare, Wetransfer, Goodreads, and even XVideos [yep, xvideos… imagine the scalability]). Below, I will write some points to consider about Ruby on Rails, in no particular order of importance.
1. It's written in Ruby
Ruby is an interpreted programming language, primarily object-oriented, that strives to resemble natural language. In Ruby, literally EVERYTHING is an object. Even data types that one might think are primitive, like numbers, are objects and it's possible to call methods on them. The way Ruby is written is very easy to understand. An example of this is the following line of code: 5.times { print "We love Ruby!" }
which means print "We love Ruby!" five times.
2. It follows the DRY principle
DRY (Don’t Repeat Yourself) literally means “Don’t repeat yourself” (though it’s more accurately interpreted as “Don’t do something you’ve already done”). It is based on reusing your code whenever possible, instead of writing it again for different, very similar cases. A notable example of this is the principle of Fat Model, Skinny Controller, which refers to writing the methods that an entity has in its model, and only executing them in the controller. Additionally, Rails uses a templating system for views, where you can write HTML with embedded Ruby, and it’s possible to create partials, which — as the name implies — are partial views that can be reused in multiple places. For example, a row with the preview of an article within a list of articles. In that case, it wouldn’t make sense to copy and paste the row’s code while changing its content; instead, the element is extracted into a partial and dynamically called whenever needed, with different content each time.
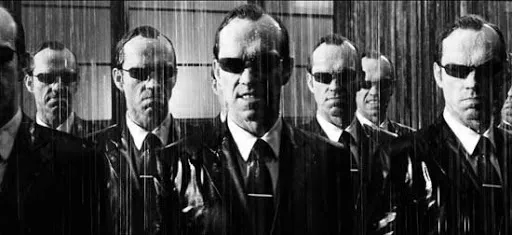
3. Convention over Configuration
Rails follows the philosophy of Convention over Configuration, and it has a special way of naming classes, methods, and files, so that explicit relationships between them (i.e., with code) are not necessary for it to internally understand their connections. For example, the previous scaffold command (rails g scaffold article title:string body:text) creates a model named Article, a controller named ArticlesController, and a folder with views named 'articles' (if the word were blog_articles, it would be translated to BlogArticles as the class name. Rails uses snake_case [separating words with underscores] for naming its methods, variables, and using generators). Additionally, each method within the controller (called an action in Rails) has an associated html.erb file with the name of that action. That is, if the controller has an index action (described as ArticlesController#index), the view that renders that method will be '/app/views/articles/index.html.erb'. In general, controllers are written in plural, and models in singular. And as if that weren't enough, if there were a relationship between a Comment model and an Article model (such as an article having comments), it would be possible to access the comments of the article object, of the Article class, simply by doing article.comments (the level of abstraction of the data layer that Rails has, called Active Record, is something that impressed me a lot).
4. The community is very active
Rails has a very large and active community, always ready to solve any problems you may encounter. Additionally, its documentation is excellent (Rails Guides). "There must be a better way to do what I'm doing" is something I've thought many times, and it's almost always true. Expert Rails users always have a very idiomatic way of writing Ruby, and something that would normally be written in 4 or 5 lines can be written in just one, without sacrificing readability. Additionally, there are many gems (which are libraries, plugins, add-ons, gadgets, or whatever you want to call them) that greatly facilitate development and provide very idiomatic methods, such as Time.now.beginning_of_day, which literally gives you the date and time the current day begins. Some notable gems include Devise (which generates a user system), Spree (for e-commerce), Rspec (for testing), and Business Time (for date calculations).
5. Getting started with Rails is easy
Ruby is a simple and very powerful language, with many methods that abstract complex logic blocks. Rails is installed as a gem after installing Ruby, and when generating a new application, it automatically creates the entire file structure to start developing. It is advisable to install Ruby using RVM (Ruby Version Manager), which allows you to install different versions of Ruby without conflicts between them, as well as install different sets of gems. In other words, you could have several versions of Rails installed for different versions of Ruby, and you could select which one you want to work with. The combination of an idiomatic language, a powerful framework with generators that do the work for you, and easy access to knowledge and source code of Rails makes RoR a particularly good choice for those who want to follow the path of agility.
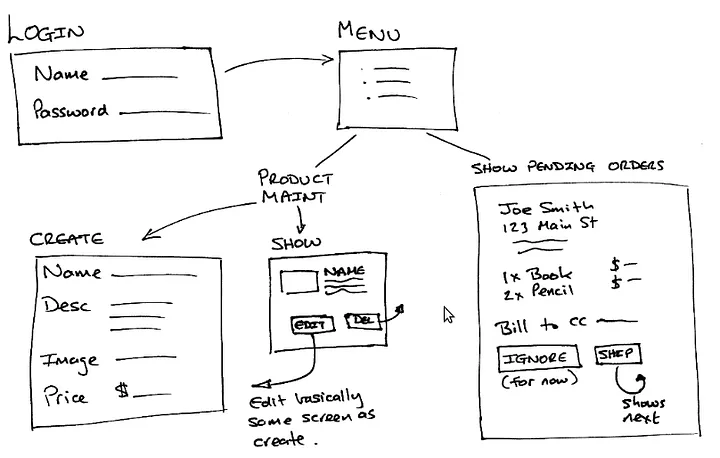
I'm sure this article left many more questions than answers, and that's because Rails has associated too many concepts due to the plethora of tools that make your work easier. However, once you understand the basics, development becomes quite straightforward. Install Ruby with RVM, install Rails, dive into the official documentation, and try it out yourself!
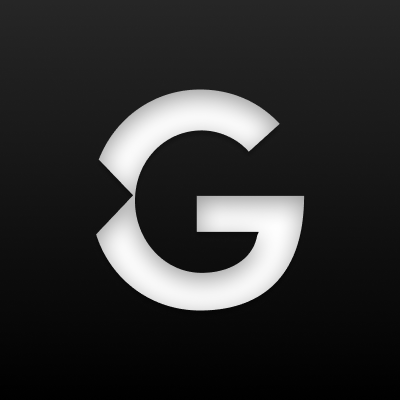
Garage Labs
Official publications from the technology consulting firm Garage Labs | Santiago, Chile